Golang+Angular+MariaDB #21 Angular Validators
Development/Go+Angular+MariaDB 2021. 2. 25. 12:51 |참고 페이지 : #삽질 없는 개발은 없다
참고 페이지 : #Angular
Input Form에 대한 검증하는 기능을 추가하겠습니다.
총 3개의 Input이 있는데, 입력 값에 따라 Issue를 보여줄 수 있도록 합니다.
src/app/app.module.ts 수정
작업에 앞서서 먼저 Module를 추가해줍니다.
...
import { FormsModule, ReactiveFormsModule } from '@angular/forms'; // 코드 수정
...
const appRoutes : Routes = [
...
]
@NgModule({
declarations: [
...
],
imports: [
...
ReactiveFormsModule, // 코드 추가
...
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule {
...
}
src/app/user/user.component.ts 수정
...
import { FormGroup, FormBuilder, Validators } from '@angular/forms'; // 코드 수정
...
@Component({
...
})
export class UserComponent implements OnInit {
...
complexForm : FormGroup; // 코드 추가
constructor(
public service: UserService,
private toastr: ToastrService,
private fbuilder : FormBuilder // 코드 추가
) {
this.service.getUsers()
.subscribe((data) => {
...
})
this.service.selectedUser = {
...
};
// 코드 추가
// 필드 당 여러개의 유효성 검사기 추가.
// 두개 이상 검사하려면 Validators.complose 함수로 검사 항목을 맵핑해야함
this.complexForm = fbuilder.group({
"name": [
null,
Validators.compose([
Validators.required, // 입력 여부 체크
Validators.minLength(2), // 최소 글자수 2개
Validators.maxLength(20), // 최대 글자수 20개
Validators.pattern('[ㄱ-ㅎ|가-힣|a-z|A-Z|0-9|\*]+$') // 한글, 영문(대소문자), 숫자 포함 여부 체크
])
],
"email": [
null,
Validators.compose([
Validators.required, // 입력 여부 체크
Validators.email // 이메일 형식 체크
])
],
"password": [
null,
Validators.compose([
Validators.required, // 입력 여부 체크
// 영문(대소문자), 숫자, 특수문자가 각각 1개 이상 포함하여야 하며, 6글자 이상 입력 여부 체크
Validators.pattern('(?=.*[a-zA-Z])(?=.*[0-9])(?=.*[!@#$%^*+=-]).{6,}$')
])
]
})
}
ngOnInit(): void {
}
...
}
Validators 함수 (링크)
class Validators {
static min(min: number): ValidatorFn
static max(max: number): ValidatorFn
static required(control: AbstractControl): ValidationErrors | null
static requiredTrue(control: AbstractControl): ValidationErrors | null
static email(control: AbstractControl): ValidationErrors | null
static minLength(minLength: number): ValidatorFn
static maxLength(maxLength: number): ValidatorFn
static pattern(pattern: string | RegExp): ValidatorFn
static nullValidator(control: AbstractControl): ValidationErrors | null
static compose(validators: ValidatorFn[]): ValidatorFn | null
static composeAsync(validators: AsyncValidatorFn[]): AsyncValidatorFn | null
}
src/app/user/user.component.html 수정
<div class="container">
<header>
...
</header>
<hr>
<div class="row">
<div class="col-md-12">
<h3>Insert User Data</h3>
<!-- 코드 수정 -->
<form method="post" #form="ngForm" [formGroup]="complexForm" (ngSubmit)="onSubmit(form)">
<input type="hidden" name="id" [(ngModel)]="service.selectedUser.id">
<div class="form-group">
<label for="name">Name : </label>
<!-- 코드 수정 -->
<input type="text" name="name" id="name" class="form-control" placeholder="insert your name"
[(ngModel)]="service.selectedUser.name" [formControl]="complexForm.controls['name']">
<!-- 코드 추가 -->
<div *ngIf="complexForm.controls['name'].touched && !complexForm.controls['name'].valid" class="alert alert-danger">
<div *ngIf="complexForm.controls['name'].hasError('required')" >필수 입력사항입니다.</div>
<div *ngIf="complexForm.controls['name'].hasError('pattern')" >특수문자 및 숫자를 입력하실 수 없습니다.</div>
<div *ngIf="complexForm.controls['name'].hasError('minlength') || complexForm.controls['name'].hasError('maxlength')" >2~20자 이내로 입력해주세요.</div>
</div>
</div>
<div class="form-group">
<label for="email">Email : </label>
<!-- 코드 수정 -->
<input type="text" name="email" id="email" class="form-control" placeholder="insert your email"
[(ngModel)]="service.selectedUser.email" [formControl]="complexForm.controls['email']">
<!-- 코드 추가 -->
<div *ngIf="complexForm.controls['email'].touched && !complexForm.controls['email'].valid" class="alert alert-danger">
<div *ngIf="complexForm.controls['email'].hasError('required')" >필수 입력사항입니다.</div>
<div *ngIf="complexForm.controls['email'].hasError('email')" >이메일 형식으로 입력해 주세요.</div>
</div>
</div>
<div class="form-group">
<label for="password">Password : </label>
<!-- 코드 수정 -->
<input type="text" name="password" id="password" class="form-control" placeholder="insert your password"
[(ngModel)]="service.selectedUser.password" [formControl]="complexForm.controls['password']">
<!-- 코드 추가 -->
<div *ngIf="complexForm.controls['password'].touched && !complexForm.controls['password'].valid" class="alert alert-danger">
<div *ngIf="complexForm.controls['password'].hasError('required')" >필수 입력사항입니다.</div>
<div *ngIf="complexForm.controls['password'].hasError('pattern')" >영문(대소문자), 숫자, 특수문자(!@#$%^*+=-)를 조합하여 8자리 이상 입력해주세요.</div>
</div>
</div>
<div class="form-row">
<div class="d-grid gap-2 d-md-flex justify-content-md-end">
<!-- 코드 수정 -->
<button class="btn btn-sm btn-block btn-primary col-lg-2" [disabled]="!complexForm.valid">
submit <fa-icon icon="user-plus"></fa-icon>
</button>
<button class="btn btn-sm btn-block btn-secondary col-lg-2" (click)="clearForm()">
clear <fa-icon icon="undo"></fa-icon>
</button>
</div>
</div>
</form>
</div>
</div>
<div class="row">
...
</div>
<div class="modal fade" id="exampleModal" tabindex="-1" aria-labelledby="exampleModalLabel" aria-hidden="true">
...
</div>
<br>
<footer>
...
</footer>
</div>
실행화면
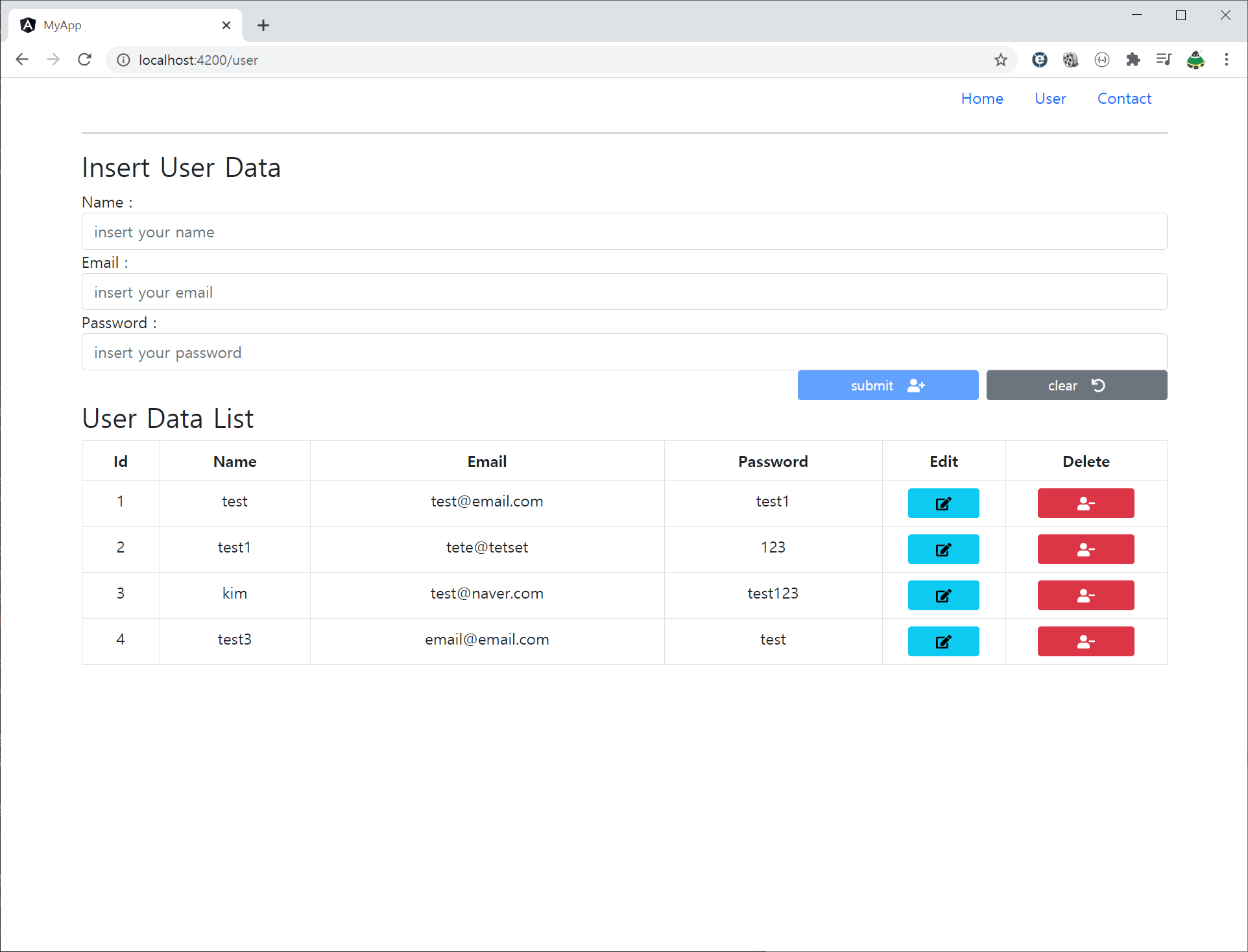
초기 실행화면에서는 입력값에 대해 Required Error가 발생되었있기에,
submit 버튼이 비활성화 상태로 되어있습니다.
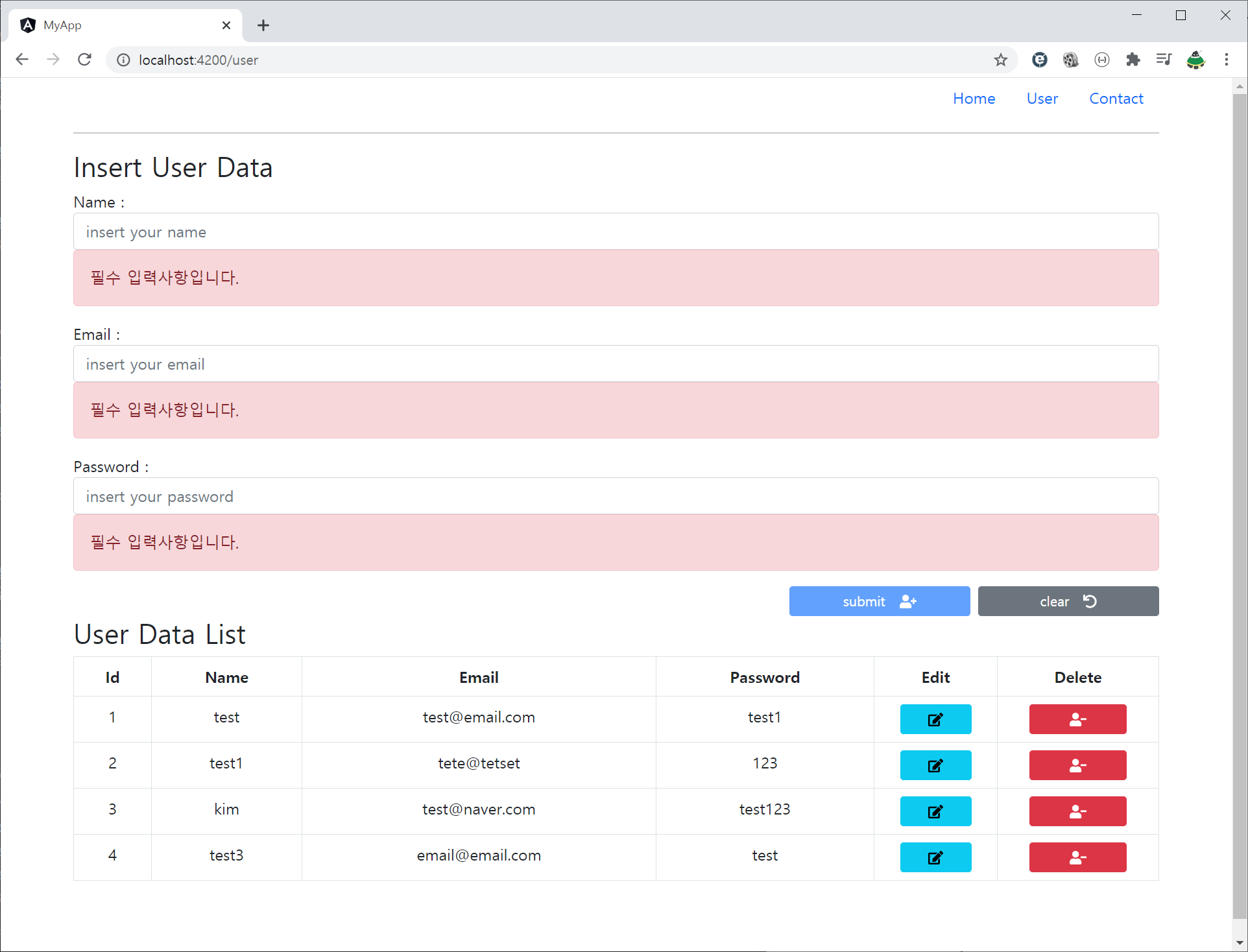
입력폼을 가볍게(?) 터치하고 넘어가면, Required Error로 인해,
문구가 표시되는 것을 볼 수 있습니다.
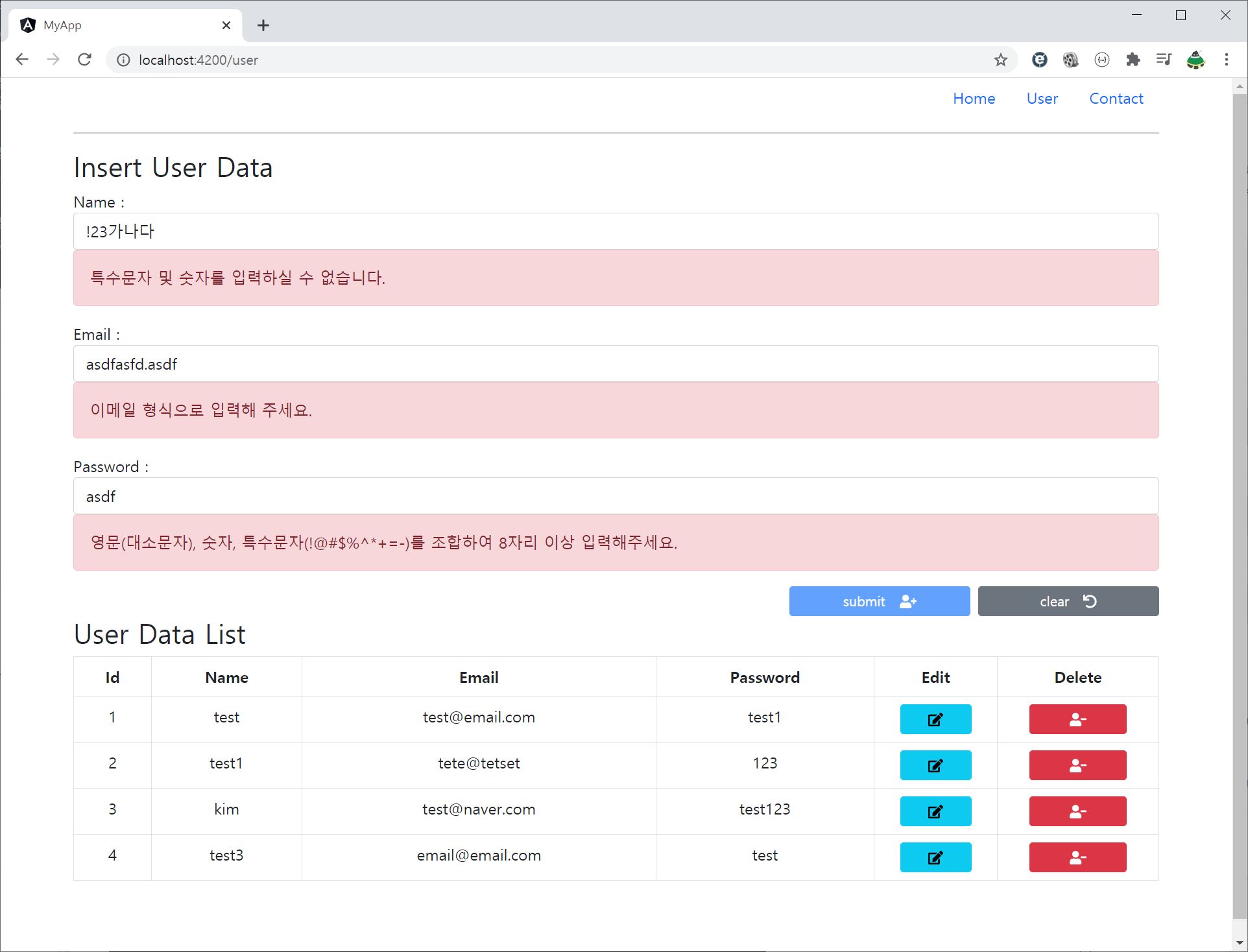
각각의 입력폼에 대한 Error로 표시되는 문구가 다르게 표시되는 이유는,
user.component.html에서 <div> 태그 내 *ngIf를 다르게 지정했기 때문입니다.
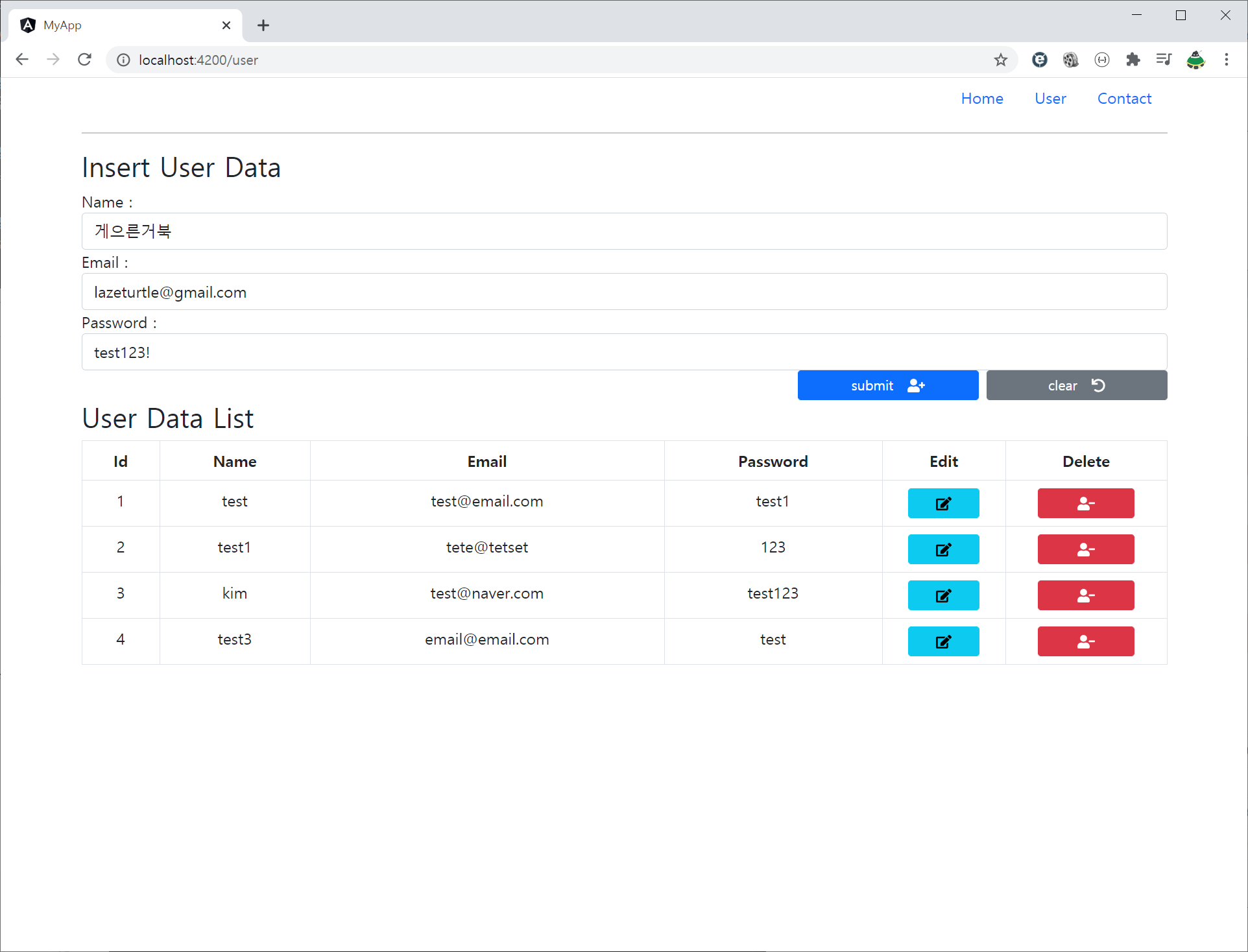
입력에 대한 검증에 맞추어 잘 입력해주시면,
Issue 문구도 사라지고, submit 버튼도 활성화 되어집니다.
'Development > Go+Angular+MariaDB' 카테고리의 다른 글
Golang+Angular+MariaDB #20 Router 기능 추가 (0) | 2021.02.15 |
---|---|
Golang+Angular+MariaDB #19 Database CRUD 추가 (0) | 2021.02.09 |
Golang+Angular+MariaDB #18 MariaDB 연동 (0) | 2021.02.08 |
Golang+Angular+MariaDB #17 Toast 띄우기 (0) | 2021.02.04 |
Golang+Angular+MariaDB #16 Bootstrap Modal 추가 + Source 보완 (0) | 2021.02.04 |